Migrate to AssertJ from Hamcrest
In this tutorial, we'll use OpenRewrite to perform an automated migration from Hamcrest to AssertJ. AssertJ is more actively maintained than Hamcrest and is generally favored for testing assertions.
Configuration
Configure your repository according to the instructions on the reference page. This is also where you'll find the full list of changes this recipe will make.
Before and After
Example test
Before
import org.junit.jupiter.api.Test;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.equalTo;
public class BiscuitTest {
@Test
public void testEquals() {
Biscuit theBiscuit = new Biscuit("Ginger");
Biscuit myBiscuit = new Biscuit("Ginger");
assertThat(theBiscuit, equalTo(myBiscuit));
assertThat("chocolate chips", theBiscuit.getChocolateChipCount(), equalTo(10));
assertThat("hazelnuts", theBiscuit.getHazelnutCount(), equalTo(3));
}
@Test
public void otherExamples() {
// ...
assertThat(foo.get("id")).isEqualTo("test-data");
assertThat(bar.size()).isEqualTo(1);
assertThat(bash.get("one")).isEqualTo(1);
assertThat(baz.size()).isEqualTo(concurrency * maxPerTask);
}
}
After
import org.junit.jupiter.api.Test;
import static org.assertj.core.api.Assertions.assertThat;
public class BiscuitTest {
@Test
public void testEquals() {
Biscuit theBiscuit = new Biscuit("Ginger");
Biscuit myBiscuit = new Biscuit("Ginger");
assertThat(theBiscuit).isEqualTo(myBiscuit);
assertThat(theBiscuit.getChocolateChipCount()).as("chocolate chips").isEqualTo(10);
assertThat(theBiscuit.getHazelnutCount()).as("hazelnuts").isEqualTo(3);
}
@Test
public void otherExamples() {
// ...
assertThat(foo).containsEntry("id", "test-data");
assertThat(bar).hasSize(1);
assertThat(bash).containsEntry("one", 1);
assertThat(baz).hasSize(concurrency * maxPerTask);
}
}
See how this recipe works across multiple open-source repositories
Run this recipe on OSS repos at scale with the Moderne SaaS.
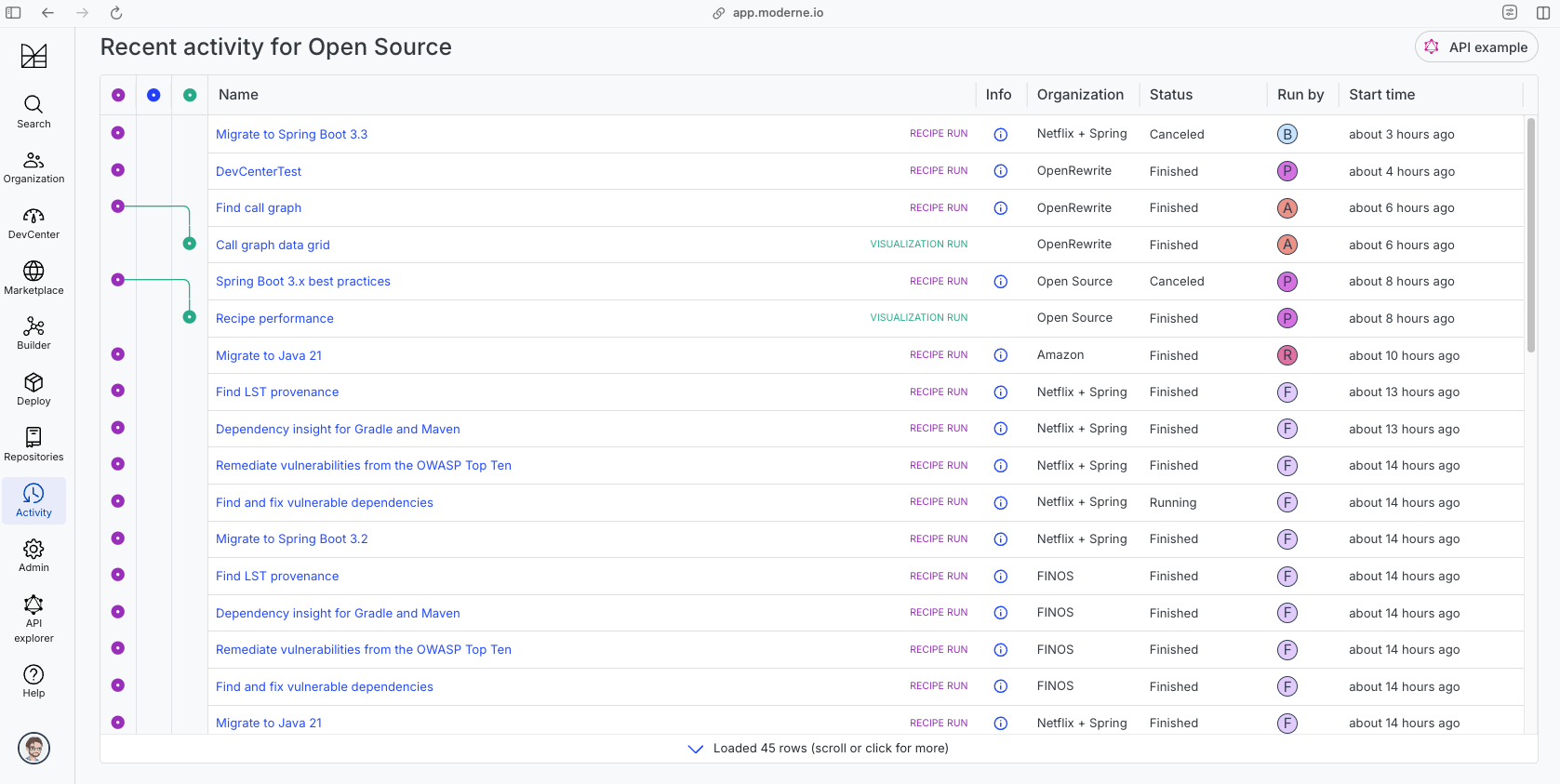
The community edition of the Moderne platform enables you to easily run recipes across thousands of open-source repositories.
Please contact Moderne for more information about safely running the recipes on your own codebase in a private SaaS.